Implementing the Entropy language by abusing decorators
The esoteric programming language entropy is a quirky experiment in giving up control. Its a language who's representation of data becomes more corrupted the more you read from it. That is to say that var foo = 5
will only equal 5 so long as you never access foo
, but the instant you do foo
is irreparably corrupted and its original value cannot be recovered. Moreover any variable you access becomes more and more noisy the more you read from it.
Absurd, right?
As with all absurd things, its possible to bring this behavior to javascript. This time its done by abusing decorators.
Checkout entropy-lang on GitHub.
While decorators are still black magic experimental, they can be used through a babel transform. After that transform is set up, implementing entropy is a trivial process.
import {number} from 'entropy-lang';
class NumTest {
@number
foo() {
return 10;
}
}
var nt = new NumTest();
// every foo is called the returned value is modified
nt.foo(); // 5.3325573585461825
Foo is:
The same thing can be done with strings as well, resulting in some unreadable mess pretty quickly.
import {str} from 'entropy-lang';
class StringTest {
@str
bar() {
return 'Hello World!';
}
}
Bar is:
After reading from these variables just a few times their original value is lost and all that is left is a mess.
So what happens when this program sings 99 bottles of beer?
At first everything starts out somewhat close to our original song.
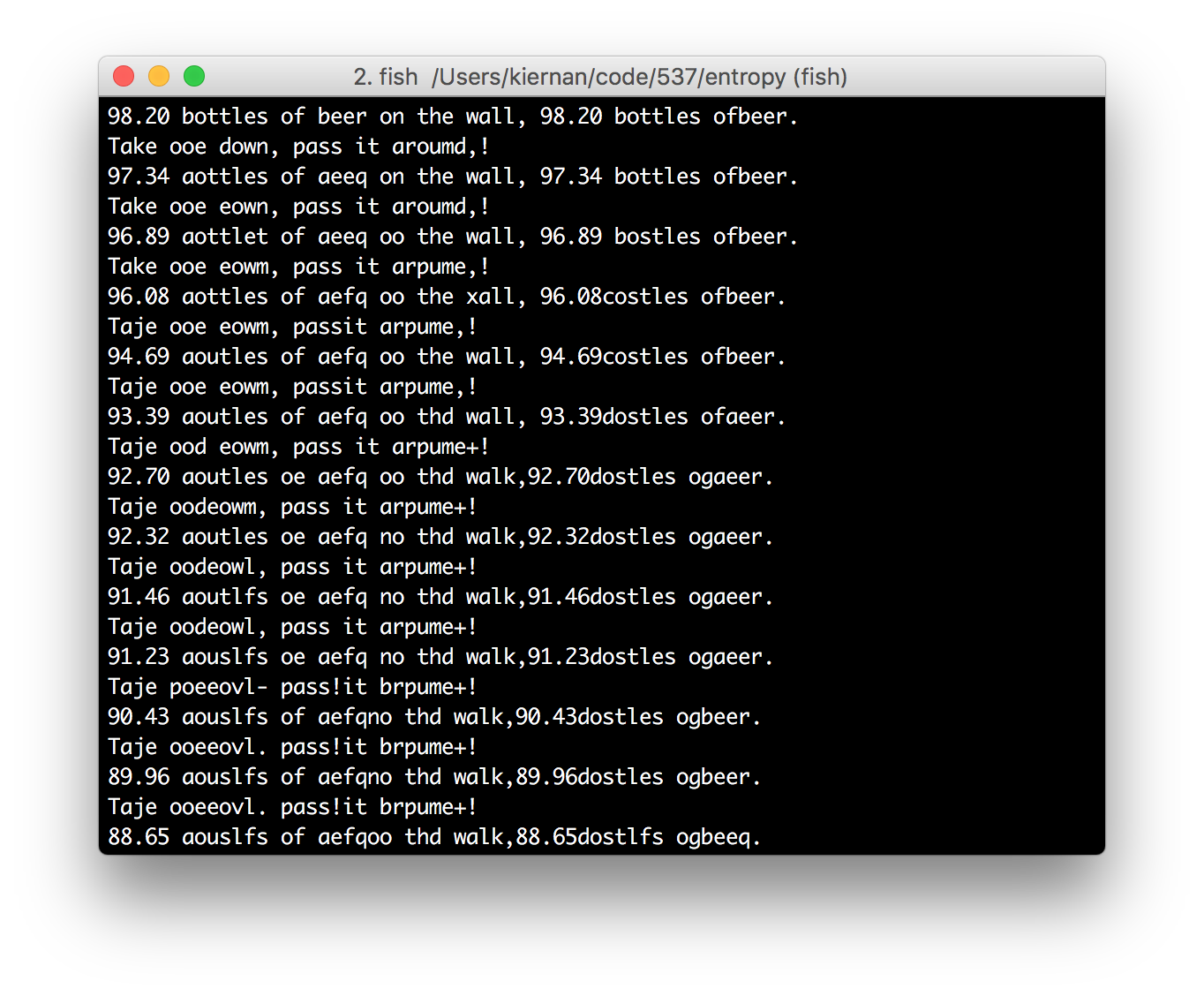
But after the 99th beer our program is clearly drunk.
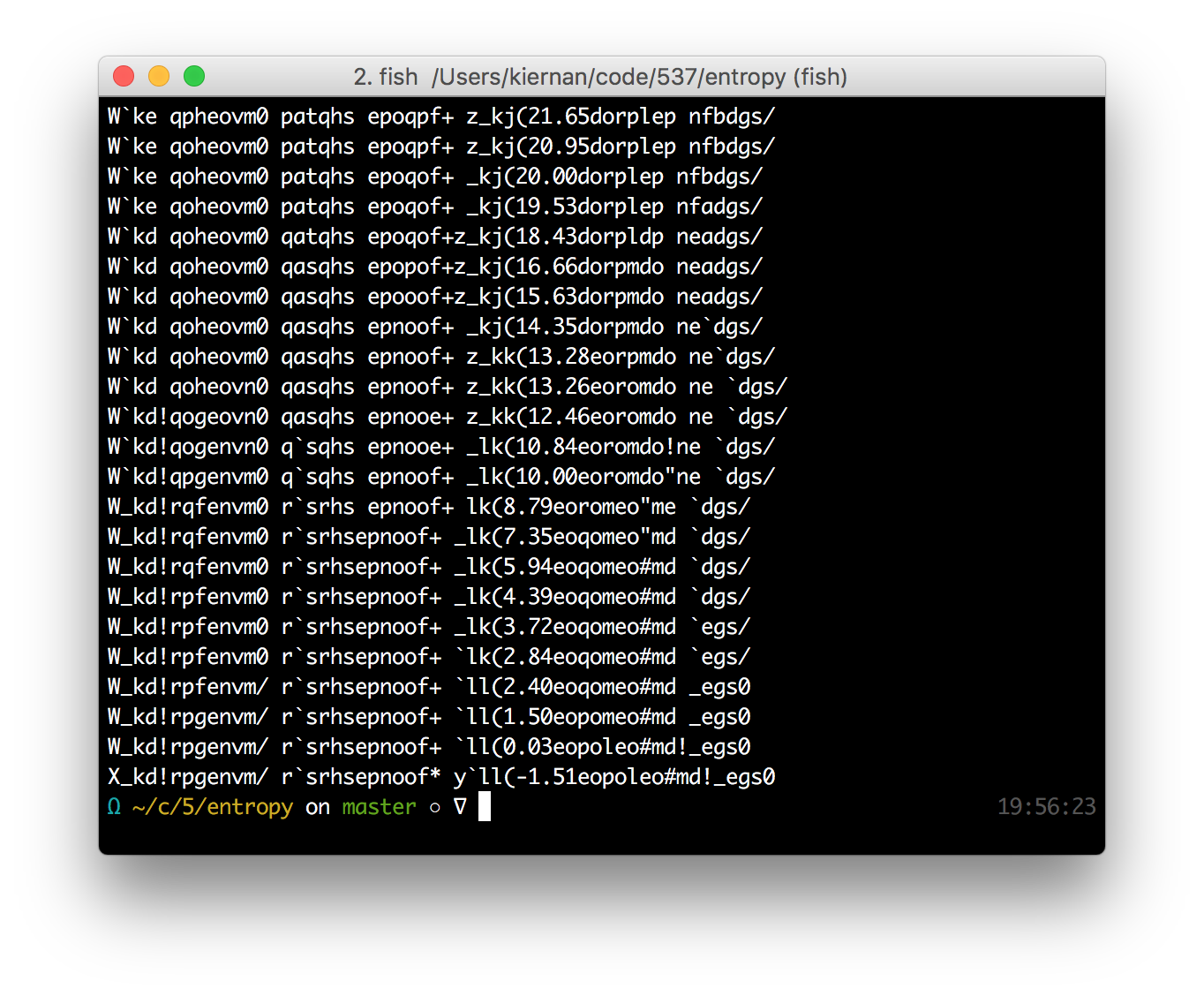
While this is nothing more than a silly experiment into what javascript and transpile magic is capable of doing today, it does show off some of the exciting possibilities in javascript's future.
Arbitrary complexity, in this case a martingale stochastic processes, can be directly attached the data that underpins our applications. Imagine being able to attach @low_pass
, @notch
, or @kalman
filters to getter functions of noisy data, cleaning up the noise with little developer interaction. This would create more reliable sources of information to make decisions on for little developer effort.